Ultimate Guide to Preventing Brute Force Attacks in Node.js
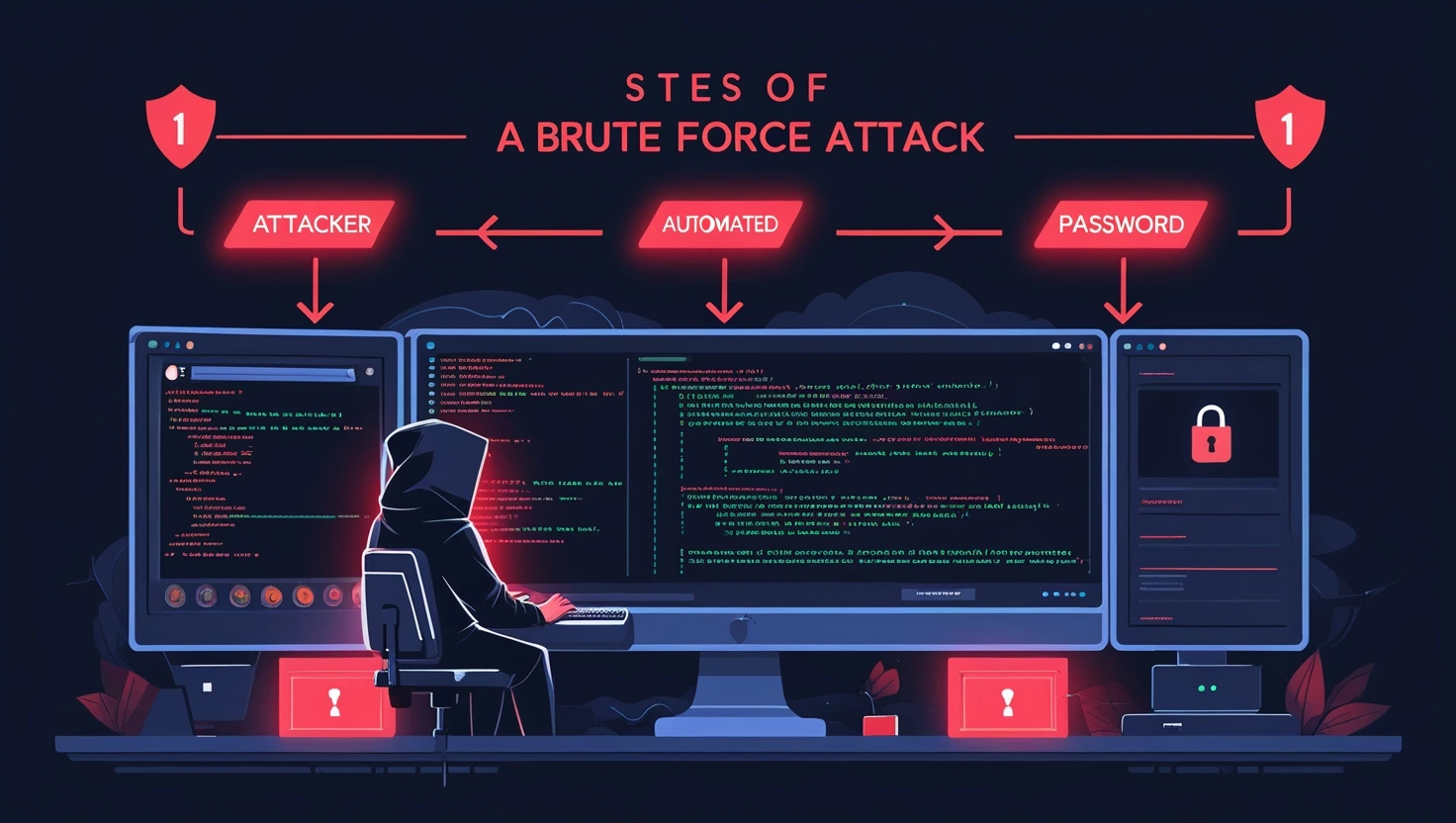
Introduction
Brute force attacks are a serious cybersecurity threat where attackers attempt to gain unauthorized access by systematically guessing usernames and passwords. Protecting your Node.js applications from these attacks is crucial to maintaining security, safeguarding user data, and ensuring application uptime.
In this comprehensive guide, we will explore powerful strategies and best practices to mitigate brute force attacks and enhance the security of your Node.js applications.
What is a Brute Force Attack?
A brute force attack is an automated process where attackers repeatedly try different password or API key combinations until they gain access. These attacks are common against:
- Login pages
- API authentication endpoints
- Admin panels
- Sensitive user accounts
If left unchecked, brute force attacks can lead to data breaches, service downtime, account takeovers, and significant financial losses.
How to Prevent Brute Force Attacks in Node.js
Implementing multiple layers of security is key to defending against brute force attacks. Below are some highly effective techniques to protect your Node.js application.
1. Implement Rate Limiting
Rate limiting restricts the number of requests an IP address can make within a specific time frame, throttling repeated login attempts.
Using express-rate-limit
const rateLimit = require('express-rate-limit'); const loginLimiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 5, // Limit each IP to 5 login attempts per window message: "Too many login attempts. Please try again later." }); app.post('/login', loginLimiter, (req, res) => { // Handle login request });
2. Monitor and Get Alerts Using Watchlog
Integrating Watchlog.io allows you to track attack attempts in real-time and receive instant alerts.
const watchlog = require("watchlog-metric"); const rateLimit = require('express-rate-limit'); const loginLimiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 5, // Limit each IP to 5 login attempts per window handler: (req, res, next) => { watchlog.increment(`rate_limit_exceeded`); res.status(429).json({ message: "Too many login attempts. Please try again later." }); } }); app.post('/login', loginLimiter, (req, res) => { // Handle login request });
With Watchlog, you gain visibility into attack patterns, enabling you to take proactive security measures.
3. Implement Account Locking with Redis
Locking accounts after repeated failed login attempts adds another layer of security.
Example using Redis:
const redis = require('redis'); const client = redis.createClient(); app.post('/login', async (req, res) => { const { username } = req.body; const key = `failed_attempts:${username}`; let attempts = await client.get(key) || 0; if (attempts >= 5) { return res.status(429).json({ message: "Account locked. Try again later." }); } const isAuthenticated = authenticateUser(username, req.body.password); if (!isAuthenticated) { await client.incr(key); await client.expire(key, 900); // Lock for 15 minutes return res.status(401).json({ message: "Invalid credentials" }); } await client.del(key); // Reset on successful login res.json({ message: "Login successful" }); });
4. Enable CAPTCHA to Block Bots
Using CAPTCHA prevents automated brute force attacks.
Google reCAPTCHA Example:
const axios = require('axios'); app.post('/login', async (req, res) => { const { captchaToken } = req.body; const secretKey = "YOUR_SECRET_KEY"; const verificationURL = `https://www.google.com/recaptcha/api/siteverify?secret=${secretKey}&response=${captchaToken}`; const response = await axios.post(verificationURL); if (!response.data.success) { return res.status(400).json({ message: "CAPTCHA verification failed" }); } // Proceed with authentication });
5. Require Strong Passwords & Multi-Factor Authentication (MFA)
- Use strong password policies with password strength checkers like
zxcvbn
. - Enable MFA (e.g., Google Authenticator, SMS verification).
6. Monitor & Log Suspicious Activity Using Watchlog
Instead of traditional logging methods, Watchlog.io provides a powerful security dashboard to track login attempts and flag suspicious activity.
Example:
const watchlog = require("watchlog-metric"); app.post('/login', (req, res) => { watchlog.increment(`login_attempts`); // Handle authentication });
7. Secure Authentication Methods
- Use bcrypt for password hashing.
- Implement OAuth or JWT-based authentication.
- Never store passwords in plain text.
Example of Secure Password Hashing:
const bcrypt = require('bcrypt'); async function hashPassword(password) { const salt = await bcrypt.genSalt(10); return await bcrypt.hash(password, salt); } async function verifyPassword(password, hash) { return await bcrypt.compare(password, hash); }
Conclusion
Brute force attacks are a serious threat, but with the right security practices, you can protect your Node.js applications effectively.
🔹 Use rate limiting and account locking
🔹 Integrate Watchlog.io for real-time monitoring
🔹 Implement MFA and strong password policies
🔹 Use CAPTCHA and secure authentication methods
Secure Your Node.js App Today! 🚀
For enterprise-level security insights, sign up on Watchlog.io and start monitoring threats in real-time!